Trong bài này mình sẽ hướng dẫn các bạn thành phần để hiện thị thông báo trong android đó là dialog và notification và thành phần để cài đặt thời gian và ngày là TimePickerDialog và DatePickerDialog.
Để các bạn hiểu rõ về các thành phần này mình sẽ làm một ứng dụng đơn giản với các button. khi click vào các button sẽ show các loại dialog tương ứng.
để làm được ví dụ này các bạn làm lần lượt theo các bước sau
Bước 1: mở file res->values->String.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">AlertDialogDemo</string>
<string name="action_settings">Settings</string>
<string name="hello_world">Hello world!</string>
<string name="AlertDialog">Alert Dialog</string>
<string name="ProgessDialog">Progess Dialog</string>
<string name="TimePicker">Time Picker</string>
<string name="DatePicker">Date Picker</string>
<string name="ShowNotification">Show Notification!</string>
</resources>
Bước 2: mở file res->layout-> activity_main.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:paddingBottom="16dp"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:paddingTop="16dp"
tools:context=".MainActivity" >
<Button
android:id="@+id/alertDialog"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:onClick="alertDialog"
android:text="@string/AlertDialog" />
<Button
android:id="@+id/progessDialog"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:onClick="progessDialog"
android:text="@string/ProgessDialog" />
<Button
android:id="@+id/timePicker"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:onClick="timePicker"
android:text="@string/TimePicker" />
<Button
android:id="@+id/datePicker"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:onClick="datePicker"
android:text="@string/DatePicker" />
<Button
android:id="@+id/showNotifi"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:onClick="showNotifi"
android:text="@string/ShowNotification" />
</LinearLayout>
Bước 3: Mở file MainActivity.java
package truong.hut.com;
import java.util.Calendar;
import android.app.Activity;
import android.app.AlertDialog;
import android.app.DatePickerDialog;
import android.app.DatePickerDialog.OnDateSetListener;
import android.app.Notification;
import android.app.NotificationManager;
import android.app.PendingIntent;
import android.app.ProgressDialog;
import android.app.TimePickerDialog;
import android.app.TimePickerDialog.OnTimeSetListener;
import android.content.Context;
import android.content.DialogInterface;
import android.content.DialogInterface.OnClickListener;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.View;
import android.widget.DatePicker;
import android.widget.TimePicker;
public class MainActivity extends Activity {
private static final String TAG = "MainActivity";
private Notification notification;
private NotificationManager notificationManager;
private int idNotification = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void alertDialog(View v) {
// b1: khoi tao alertdialoe
final AlertDialog.Builder builder = new AlertDialog.Builder(this);
// b2: xay dung khung cho alertdialog
// thiet lap icon
builder.setIcon(this.getResources().getDrawable(R.drawable.ic_launcher));
// builder.setIcon(R.drawable.ic_launcher);
// thiet lap tieu de
builder.setTitle("Alert Dialog");
builder.setTitle(getResources().getString(R.string.action_settings));
// thiet lap noi dung
builder.setMessage("Are you sure you want to exit?");
// thiet lap nut hien thi cho dialog
builder.setPositiveButton("OK", new OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
Log.d(TAG, "onclick button OK");
finish();
}
});
builder.setNeutralButton("AAA", new OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
Log.d(TAG, "onclick button AAA");
dialog.dismiss();
}
});
builder.setNegativeButton("BBB", new OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO Auto-generated method stub
Log.d(TAG, "onclick button BBB");
}
});
builder.show(); // hien thi len man hinh
}
// tuong tu xay dung dialog voi progessDialog, timePicker, datePicker
public void progessDialog(View v) {
final ProgressDialog progessDialog = new ProgressDialog(this);
progessDialog.setIcon(this.getResources().getDrawable(
R.drawable.ic_launcher));
progessDialog.setTitle("ProgessDialog");
progessDialog.setMessage("Loading...");
progessDialog.setCanceledOnTouchOutside(true);
// cac ban co the add them cac button cho progressDialog theo src duoi day
// thong thuong progressdialog se hien thi khi download nen se duoc dismiss khi download ket thuc
// progessDialog.setButton("OK", new DialogInterface.OnClickListener() {
//
// @Override
// public void onClick(DialogInterface dialog, int which) {
// // TODO Auto-generated method stub
// Log.d(TAG, "onclick button OK");
// finish();
// }
// });
//
// progessDialog.setButton2("CANCEL",
// new DialogInterface.OnClickListener() {
//
// @Override
// public void onClick(DialogInterface dialog, int which) {
// // TODO Auto-generated method stub
// Log.d(TAG, "onclick button CANCELF");
// dialog.dismiss();
//
// }
// });
progessDialog.show();
}
public void timePicker(View v) {
// lay thoi gian cua dien thoai
Calendar calerdar2 = Calendar.getInstance();
int hourOfDay = calerdar2.get(Calendar.HOUR_OF_DAY);
int minute = calerdar2.get(Calendar.MINUTE);
boolean is24HourView = true;
TimePickerDialog timepicker = new TimePickerDialog(MainActivity.this,
new OnTimeSetListener() {
@Override
public void onTimeSet(TimePicker view, int hourOfDay,
int minute) {
// TODO Auto-generated method stub
Log.d(TAG, hourOfDay + ":" + minute);
}
}, hourOfDay, minute, is24HourView);
timepicker.show();
}
public void datePicker(View v) {
// lay ngay thang nam hien tai cua dien thoai
Calendar calendar = Calendar.getInstance();
int year = calendar.get(Calendar.YEAR);
int monthOfYear = calendar.get(Calendar.MONTH);
int dayOfMonth = calendar.get(Calendar.DAY_OF_MONTH);
DatePickerDialog datepicker = new DatePickerDialog(MainActivity.this,
new OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year,
int monthOfYear, int dayOfMonth) {
// TODO Auto-generated method stub
Log.d(TAG, dayOfMonth + "-" + monthOfYear + "-" + year);
}
}, year, monthOfYear, dayOfMonth);
datepicker.show();
}
public void showNotifi(View v) {
// b1: khoi tao NotificationManager
notificationManager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
// b2: dinh nghia notification gom co icon, time,massage
CharSequence ticker = "New Message";
int icon = R.drawable.ic_launcher;
long when = System.currentTimeMillis(); // thoi gian hien tai
notification = new Notification(icon, ticker, when);
// b3: thiet lap thong tin cua epended meassage
Context context = getApplicationContext();
CharSequence title = "Bui Son Truong";
CharSequence text = "where are you now?";
// moi khi kich vao epended message se thuc hien mot action nao do
// vi du hien thi len 1 web
Intent intent = new Intent(Intent.ACTION_VIEW,
Uri.parse("https://www.google.com.vn/"));
PendingIntent pi = PendingIntent.getActivity(context, 0, intent, 0);
notification.setLatestEventInfo(context, title, text, pi);
// thiet lap am thanh
notification.defaults |= Notification.DEFAULT_SOUND;
// notification.sound = Uri.parse("file:///sdcard/ic_hbl.mp3");
// thiet lap rung
notification.defaults |= Notification.DEFAULT_VIBRATE;
// long[] vi = { 0, 100, 0, 2000, 0, 3000, 0, 4000 };
// notification.vibrate = vi;
// thiet lap do sang
notification.defaults |= Notification.DEFAULT_LIGHTS;
// b4: Show thong bao
notificationManager.notify(idNotification, notification);
}
// public void CancelNotification(View view) {
// // thuc hien xoa thong bao
// notificationManager.cancel(idNotification);
// }
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}<span style="line-height: 1.5em;">
Note:
Các bạn có thể theo dõi kết quả thực hiện chương trình trên logcat và màn hình. . Khi làm theo ví dụ các bạn có gì không hiểu có thể để lại câu hỏi trên web mình sẽ trả lời các bạn một cách nhanh nhất có thể. Các bạn có thể down full src theo link dưới đây
AlertDialogDemo
Kết quả thực hiện chương trình
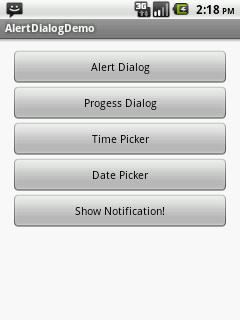
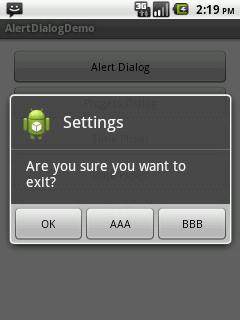
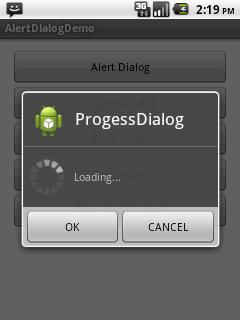
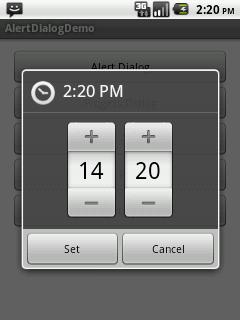
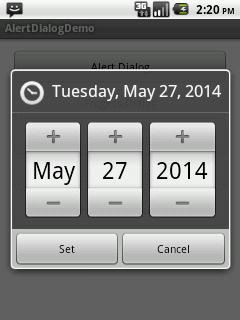
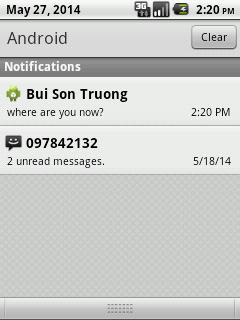