Trong bài này mình sẽ hướng dẫn các bạn cách đọc và ghi file trong android. Các bạn có thể đọc và ghi một file vào bộ nhớ trong và bộ nhớ ngoài trong android.
Để các bạn hiểu rõ phần này mình sẽ làm một ứng dụng đơn giản với các hàm đọc và ghi file trong android vào Internal Storage và External Storage ngoài ra các bạn cũng có thể đọc file từ một foder trong adroid.
Để làm được ví dụ này bạn làm lần lượt theo các bước sau
Bước 1: mở file AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.read_write_is"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="8" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<activity
android:name="com.example.read_write_is.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Note: do chúng ta cần ghi ra bộ nhớ ngoài nên cần khai báo quyền
<uses-permission android:name=”android.permission.WRITE_EXTERNAL_STORAGE” />
Bước 2: mở file res->layout-> layout_mainactivity.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity" >
<TextView
android:id="@+id/tvContentFile"
android:layout_width="fill_parent"
android:layout_height="wrap_content" />
</RelativeLayout>
Bước 3 tạo class LogUtil.java trong packet com.example.read_write_is
package com.example.read_write_is;
import android.util.Log;
public class LogUtil {
public static void LogD(String Tag, String Message) {
Log.d(Tag, Message);
}
public static void LogE(String Tag, String Message) {
Log.e(Tag, Message);
}
public static void LogI(String Tag, String Message) {
Log.i(Tag, Message);
}
public static void LogW(String Tag, String Message) {
Log.w(Tag, Message);
}
}
Bước 4 tạo foder res->raw
Copy 1 file .txt bất kỳ bạn muốn đọc nội dung trong ví dụ này mình copy file có tên cmd_find_log.txt
cmd_find_log
Bước 5: mở file MainActivity.java
package com.example.read_write_is;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import android.app.Activity;
import android.content.Context;
import android.content.res.Resources;
import android.os.Bundle;
import android.os.Environment;
import android.view.Menu;
import android.widget.TextView;
public class MainActivity extends Activity {
private static final String TAG = "MainActivity";
private static final String FILENAME = "HighScore.txt";
private static final String ContentFile = "1. TruongBS 99999 \n2. LTT 88888";
private static final String FILENAME_ES = "DS.txt";
private TextView tvContentFile;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
try {
// ghi vao file co name FILENAME = "HighScore.txt" noi dung la ContentFile
WriteToFile(FILENAME, ContentFile);
tvContentFile = (TextView) findViewById(R.id.tvContentFile);
// doc noi dung tu file co ten FILENAME va set gia tri cho textview
tvContentFile.setText(ReadFromFile(FILENAME));
// copy 1 file bat ky trong thu muc res->raw trong vi du nay minh copy
// file co ten la cmd_find_log
// doc 1 file tu foder res->raw va hien thi toan bo file ra logcat
LogUtil.LogD(TAG, ReadStaticfile(R.raw.cmd_find_log));
// ghi vao file co name FILENAME = "HighScore.txt" noi dung la ContentFile ra bo nho ngoai
// de co the ghi ra bo nho ngoai ban can khai bao trong manifest
// <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
// de co the thay duoc file trong the nho ban can khai bao dung luong trong SDcard cua may ao
WriteToFileES(FILENAME, ContentFile);
// doc 1 file tu bo nho ngoai co ten la FILENAME_ES = "DS.txt"
// de chay duoc ham nay ban phai copy 1 file co name FILENAME_ES = "DS.txt" vao bo nho ngoai
LogUtil.LogD(TAG, ReadFileFromES(FILENAME_ES));
} catch (Exception e) {
// TODO: handle exception
LogUtil.LogE(TAG, e.toString());
}
}
private String ReadFileFromES(String filenameEs) {
// TODO Auto-generated method stub
String result = null;
try {
// buoc 1 mo file
FileInputStream fis = new FileInputStream(
Environment.getExternalStorageDirectory() + "//"
+ filenameEs);
// buoc 2 doc file
int flength = fis.available();
if (flength != 0) {
byte[] buffer = new byte[flength];
if (fis.read(buffer) != -1) {
result = new String(buffer);
}
}
// buoc 3 dong file
fis.close();
} catch (Exception e) {
// TODO: handle exception
LogUtil.LogE(TAG, e.toString());
}
return result;
}
private void WriteToFileES(String fname, String fcontent) {
// TODO Auto-generated method stub
try {
// buoc 1 mo file de gi
FileOutputStream fos = new FileOutputStream(
Environment.getExternalStorageDirectory() + "//" + fname);
// buoc 2 ghi noi dung vao file
fos.write(fcontent.getBytes());
// buoc 3 dong file
fos.close();
} catch (Exception e) {
// TODO: handle exception
LogUtil.LogE(TAG, e.toString());
}
}
private void WriteToFile(String filename2, String contentfile2) {
// TODO Auto-generated method stub
try {
// buoc 1 mo file de gi
FileOutputStream fos = openFileOutput(filename2,
Context.MODE_PRIVATE);
// buoc 2 ghi noi dung vao file
fos.write(contentfile2.getBytes());
// buoc 3 dong file
fos.close();
} catch (Exception e) {
// TODO: handle exception
LogUtil.LogE(TAG, e.toString());
}
}
private String ReadFromFile(String fName) {
String result = null;
try {
// buoc 1 mo file
FileInputStream fis = openFileInput(fName);
// buoc 2 doc file
int flength = fis.available();
if (flength != 0) {
byte[] buffer = new byte[flength];
if (fis.read(buffer) != -1) {
result = new String(buffer);
}
}
// buoc 3 dong file
fis.close();
} catch (Exception e) {
// TODO: handle exception
LogUtil.LogE(TAG, e.toString());
}
return result;
}
private String ReadStaticfile(int rsID) {
String result = null;
try {
Resources resources = getResources();
InputStream is = resources.openRawResource(rsID);
// buoc 2 doc file
int flength = is.available();
if (flength != 0) {
byte[] buffer = new byte[flength];
if (is.read(buffer) != -1) {
result = new String(buffer);
}
}
} catch (Exception e) {
// TODO: handle exception
LogUtil.LogE(TAG, e.toString());
}
return result;
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
Note:
Để thực hiện được ví dụ này các bạn cần tạo một máy ảo có cả bộ nhớ trong và bộ nhớ ngoài
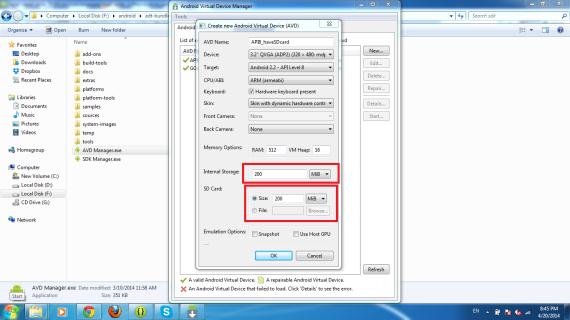
Để đọc sử dụng được hàm ReadFileFromES
các bạn cần mở DDMS -> File Explorer -> “push a file onto the device”
lưu ý rằng bạn chỉ có thể vào DDMS và push file vào khi đã chạy máy ảo và máy ảo phải có SDCard
và chọn 1 file .txt bạn muốn đọc nội dung trong ví dụ này mình tạo 1 file đơn giản là DS.txt với mục đích mô tả việc đọc một file từ SDCard và ghi ra logcat
DS
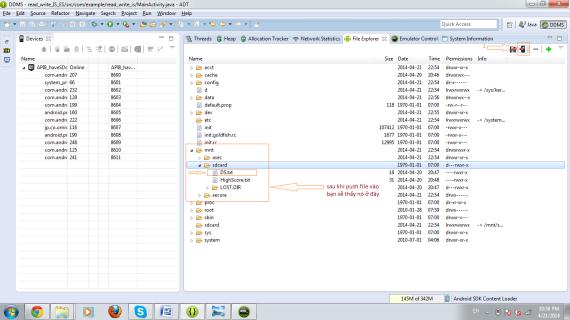
Các bạn có thể theo dõi kết quả thực hiện chương trình trên logcat và màn hình. Khi làm theo ví dụ các bạn có gì không hiểu có thể để lại câu hỏi trên web mình sẽ trả lời các bạn một cách nhanh nhất có thể. Các bạn có thể down full src theo link dưới đây
Kết quả thực hiện chương trình
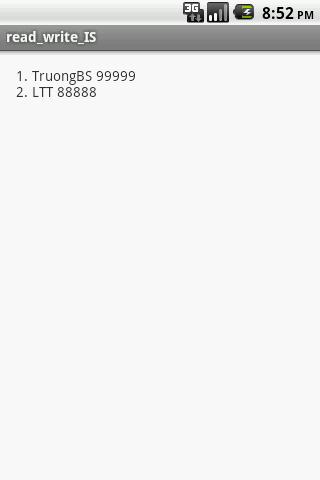
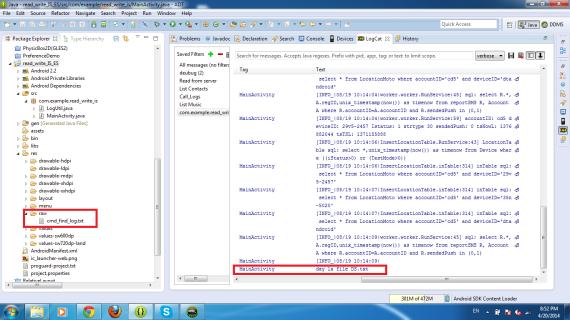
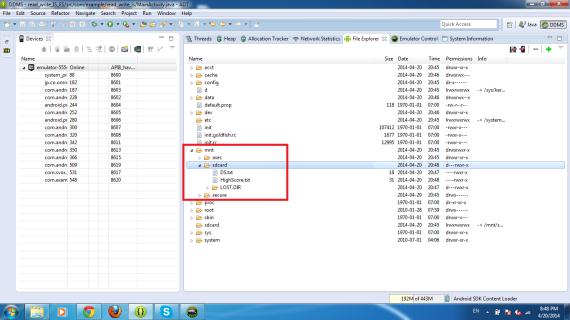
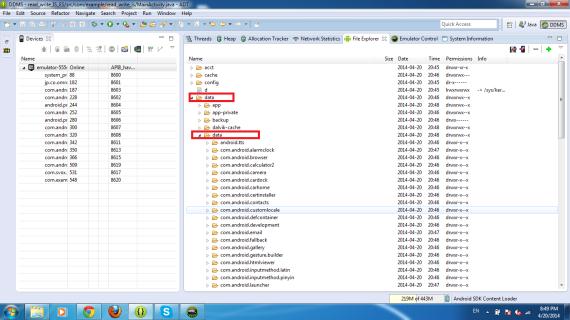
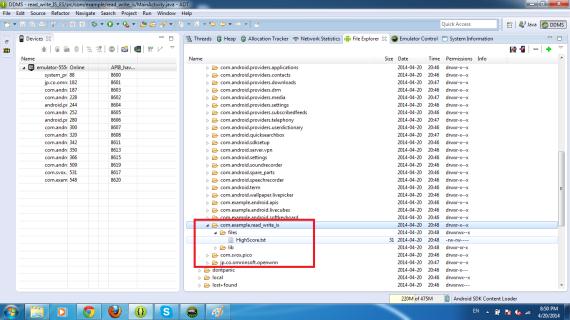