Sharepreferences là một Interface trong android giúp chúng ta có thể lưu và truy xuất dữ liệu một cách dễ dàng. Thực tế dữ liệu được lưu dưới dạng file xml với “tag” và “value”. Thông thường Sharepreferences được sử dụng rất nhiều để lưu cấu hình, lưu highscore, lưu user pass và trạng thái remmember khi login mà các bạn đã thấy rất nhiều…
Để các bạn có thể hiểu rõ về vấn đề này mình làm một ứng dụng đơn giản để lưu lại hướng màn hình, lưu lại trạng thái checkbox, khi checkbox được check thì edittext user và pass sẽ được set giá trị được remember trong file
Để làm được app này các bạn có thể thực hiện theo các sau
Bước 1: mở file res->values-> Strings.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">PreferenceDemo</string>
<string name="action_settings">Settings</string>
<string name="hello_world">Hello world!</string>
<string name="Remember">Remember</string>
<string name="user">user</string>
<string name="pass">pass</string>
<string name="Config">Config</string>
<string name="huong">nhap huong man hinh</string>
</resources>
Bước 2: mở file res->layout-> layout_mainactivity.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<EditText
android:id="@+id/etNumber"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:hint="@string/huong"
android:inputType="numberDecimal" />
<Button
android:id="@+id/btConfig"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:text="@string/Config" />
<EditText
android:id="@+id/etUser"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:hint="@string/user" />
<EditText
android:id="@+id/etPass"
android:inputType="textPassword"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:hint="@string/pass" />
<CheckBox
android:id="@+id/cbRemember"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/Remember" />
</LinearLayout>
Bước 3 tạo class Constant.java trong packet com.example.preferencedemo
package com.example.preferencedemo;
public class Constant {
public static final String REMEMBER = "REMEMBER";
public static final String ORIENTATION = "ORIENTATION";
public static final String USER = "USER";
public static final String PASS = "PASS";
public static final String MyNameFileFre = "MyPrefer";
}
Bước 4 tạo file PreferenceUtil.java trong packet com.example.preferencedemo
package com.example.preferencedemo;
import android.content.Context;
import android.content.SharedPreferences;
import android.preference.PreferenceManager;
public class PreferenceUtil {
private SharedPreferences preferences;
// xay dung phuong thuc khoi tao file preferrences mac dinh
public PreferenceUtil(Context mContext) {
preferences = PreferenceManager.getDefaultSharedPreferences(mContext);
}
// khoi tao file preference co ten la name
public PreferenceUtil(Context mContext, String name) {
preferences = mContext.getSharedPreferences(name, Context.MODE_PRIVATE);
}
// Xay dung cac phuong thuc set get cac gia tri duoc luu trong file XML
// xay dung phuong thuc set get cho orientation
public void setOrientation(int orientation) {
preferences.edit().putInt(Constant.ORIENTATION, orientation).commit();
}
public int getOrientation() {
return preferences.getInt(Constant.ORIENTATION, 0);
}
// xay dung phuong thuc set get cho Remember
public void setRemember(int remember) {
// Editor myEditor = preferences.edit();
// myEditor.putInt(Constant.REMEMBER, remember);
// myEditor.commit();
preferences.edit().putInt(Constant.REMEMBER, remember).commit();
}
public int getRemember() {
return preferences.getInt(Constant.REMEMBER, 0);
}
// xay dung phuong thuc set get cho USER
public void setUser(String mUser) {
preferences.edit().putString(Constant.USER, mUser).commit();
}
public String getUser() {
return preferences.getString(Constant.USER, "");
}
// xay dung phuong thuc set get cho PASS
public void setPASS(String mPASS) {
preferences.edit().putString(Constant.PASS, mPASS).commit();
}
public String getPASS() {
return preferences.getString(Constant.PASS, "");
}
}
Bước 5 mở file MainActivity.java
package com.example.preferencedemo;
import android.app.Activity;
import android.content.pm.ActivityInfo;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.CompoundButton.OnCheckedChangeListener;
import android.widget.EditText;
public class MainActivity extends Activity {
private PreferenceUtil pu;
private PreferenceUtil puName;
private EditText et;
private Button bt;
private CheckBox cb;
private EditText etUser,etPass;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// trong vi du nay minh huong dan cac ban 2 cach lam viec voi Preference
// dung file mac dinh pu
pu = new PreferenceUtil(this);
// dung file co ten file Constant.MyNameFileFre = MyPrefer
puName = new PreferenceUtil(this, Constant.MyNameFileFre);
// link den layout de dieu khien
et = (EditText) findViewById(R.id.etNumber);
bt = (Button) findViewById(R.id.btConfig);
etUser = (EditText) findViewById(R.id.etUser);
etPass = (EditText) findViewById(R.id.etPass);
cb = (CheckBox) findViewById(R.id.cbRemember);
// bat su kien click vao button config
bt.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
// trong vi du nay de don gian minh lam 1 editext de cac ban nhap so
// neu cac ban nhap 0 la huong theo sensor ; 1 la huong thang dung; 2 la huong ngang
String str = et.getText().toString();
if (!str.equals("")) {
// ghi gia tri huong man hinh xuong file preference
pu.setOrientation(Integer.parseInt(str));
// set huong cho man hinh theo gia tri trong file preference
setOrientation(pu.getOrientation());
}
}
});
// luu lai gia tri cua user va pass neu cb Remember duoc check tuong tu yahoo, facebook
cb.setOnCheckedChangeListener(new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
// TODO Auto-generated method stub
if (isChecked) {
// neu checkbox duoc check thi luu gia tri remember =1
// luu user va pass xuong file preference
pu.setRemember(1);
puName.setRemember(1);
String user = etUser.getText().toString();
pu.setUser(user);
String pass = etPass.getText().toString();
pu.setPASS(pass);
Log.d("debug", "Remember");
} else {
// neu checkbox khong dc check thi set gia tri remember =0
pu.setRemember(0);
puName.setRemember(0);
Log.d("debug", "Don't Remember");
}
;
}
});
}
@Override
protected void onResume() {
// TODO Auto-generated method stub
super.onResume();
// khoi tao huong cho man hinh moi khi activity duoc chay
// viec set huong nay cac ban co the thay rat nhieu trong cac game
// hoac cac app co huong man hinh mac dinh theo setting cua nguoi dung
setOrientation(pu.getOrientation());
// get lai gia tri remmember
int i = pu.getRemember();
if (i == 0) {
// don't remember user + pass
Log.d("debug", "don't remember");
// set gia tri cho checkbox la false( not check)
cb.setChecked(false);
} else {
// neu co remember user + pass thi set gia tri user + pass cho edittext va set gia tri cho checkbox = true (isCheck)
String user = pu.getUser();
etUser.setText(user);
etPass.setText(pu.getPASS());
cb.setChecked(true);
Log.d("debug", "User "+ user+" Pass "+ pu.getPASS());
}
}
private void setOrientation(int orientation) {
// phuong thuc nay de set huong cho man hinh
switch (orientation) {
case 0:
// set huong cua man hinh theo cam bien
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_SENSOR);
break;
case 1:
// set huong cua man hinh la huong thang dung
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
break;
case 2:
// set huong cua man hinh la huong ngang
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE);
break;
default:
// mac dinh de huong man hinh la thang dung
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
break;
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
Các bạn có thể xem file sharepreference theo link:
chọn DDMS -> File Exprolrer -> data -> data -> <packet – name> (như hình dưới)
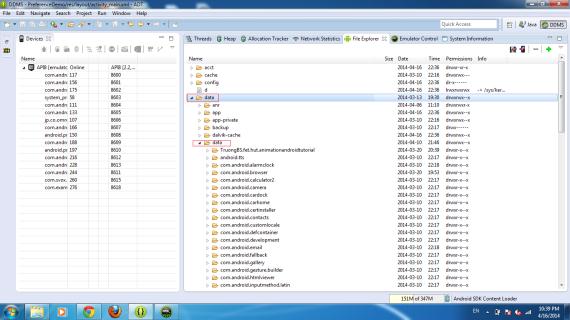
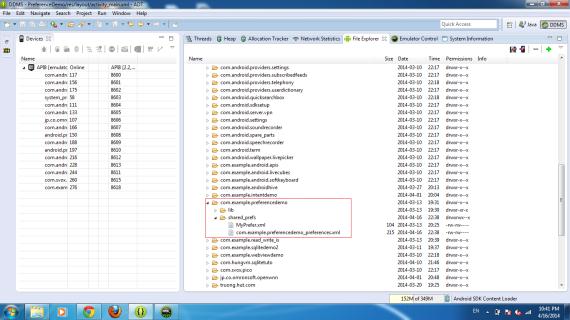
Cấu trúc file sharePreferences có dạng như hình dưới
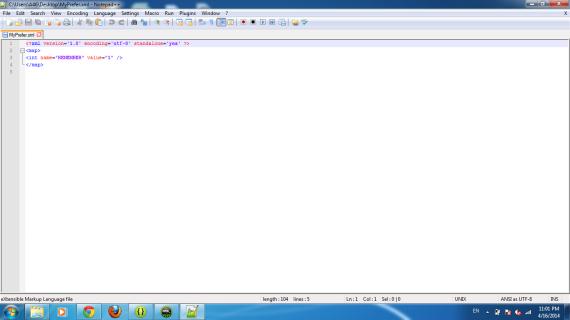
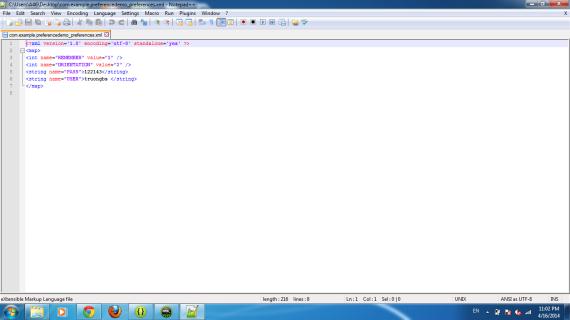
Note:Các bạn có thể theo dõi kết quả thực hiện chương trình trên logcat và màn hình. Các bạn có thể thoát app và vào lại các bạn sẽ thấy các cấu hình sẽ giống như trước khi bạn out ra khỏi app. Khi làm theo ví dụ các bạn có gì không hiểu có thể để lại câu hỏi trên web mình sẽ trả lời các bạn một cách nhanh nhất có thể. Các bạn có thể down full src theo link dưới đây
PreferenceDemo
Kết quả thực hiện chương trình
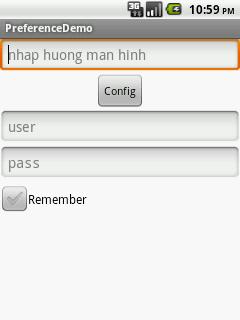
.jpg)